UPDATE: THE CONTENT OF THIS SPECIFIC ARTICLE ABOUT WEBRTC SCREEN SHARING IS OLD AND SLIGHTLY INACCURATE. PLEASE LOOK FOR ANOTHER RESOURCE AS AN EXAMPLE
After voice, video, instant messaging, screencasting, presence and sending files; the only missing piece for collaboration would be screen sharing. Here’s how you do it in WebRTC.
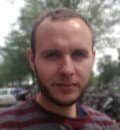
[Konstantin Goncharuk (@kkooler) is Lead Developer at Videolink2.me – a free online video calls service with instant messages and… screen sharing. He was kind enough to provide the details on how to implement screen sharing in WebRTC on Chrome.]
Last couple of years web browsers extremely extended their functions from being “viewers for web pages” to all-in-one web application frameworks. Modern web browser provides local storage, database, multi-threading, peer to peer connection and dozens of other possibilities, that give developers a chance to bring user experience from native apps to web.
WebRTC for Screen sharing
Definitely the hero of last year was WebRTC — framework that provides direct data and media stream transfer between two browsers without external server involved. It’s breakthrough for online video communication apps, since developers don’t need to support hundreds of servers that act as a bridge for user media streams.
Now they only need to exchange participants “webrtc addresses” and let them use p2p connection for data transfer.
And WebRTC is production ready! We use it as one of connection channels in Videolink2.me to provide our users with high quality video calls service in web browsers.
But what will we see with WebRTC? Maybe screen sharing?
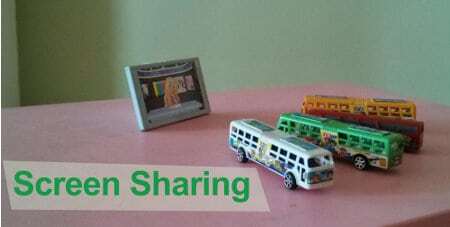
The current version of Chrome already supports plugin-free screen sharing. While there are thoughts on moving that into a mandatory Chrome extension, the implementation will probably stay the same. Why not prepare for it by implementing screen sharing?
Let’s take a look at how screen sharing works and how difficult is it to implement it in a web app.
WebRTC to share your screen
As I mentioned above, WebRTC enables video calling between two browsers. The next step for true collaboration is to enable screen sharing as well – which is exactly what Chrome’s developers decided to do.
Chrome screen sharing doesn’t allow you to manipulate the shared screen, but only view it as a video stream. And as a developer, you work with the screen’s stream the same way as you work with the web camera or microphone. And use WebRTC to transmit screen sharing the same way as usual media streams.
Interested? Let’s take a closer look.

Before developing you need to enable screen sharing: open chrome://flags/#enable-usermedia-screen-capture and enable “Enable screen capture support in getUserMedia()”.
Access to media streams
To get access to user media streams we use getUserMedia function, that takes object with description of desired stream and returns it back into callback.
That’s how it looks if you want to get user web camera with audio:
navigator.getUserMedia({audio: true, video: true}, function(stream) { //We’ve got media stream }, function() { //Error, no stream provided } )
We use the same way to get user screen but with different stream description:
navigator.getUserMedia({ audio: false, video: { mandatory: { chromeMediaSource: 'screen', maxWidth: 1280, maxHeight: 720 }, optional: [] } }, function(stream) { //We've got media stream }, function() { //Error, no stream provided } )
We asked browser to provide screen media stream with maximum size 1280×720 without audio. After executing the code above, Chrome will ask our permission to share the screen and if we agree — pass our screen capture as a media stream to the callback.
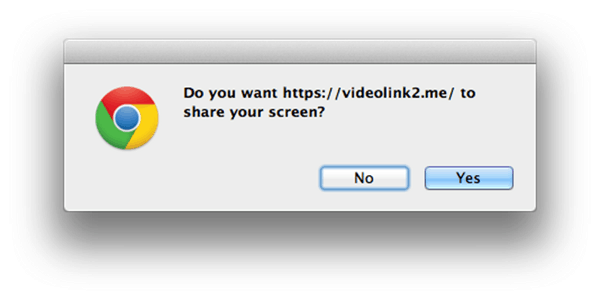
Adding Video Stream to WebRTC connection
As soon as we got it we can add to WebRTC connection as any usual video stream:
navigator.getUserMedia({ audio: false, video: { mandatory: { chromeMediaSource: 'screen', maxWidth: 1280, maxHeight: 720 }, optional: [] } }, function(stream) { pc.addStream(stream); }, function() { //Error, no stream provided } )
So the received stream can be transmitted via WebRTC the same way as web camera and easily played in <video> component on the other side.
One restriction that should be taken into consideration — screen sharing works only with SSL enabled (https://) domains.
To see it in action, let’s make a simple example that will capture she creen and play it in the video player.
Our html page will contain button to start screen sharing and video to show shared screen:
<html> <head> <title>Screen sharing example</title> <script src="https://code.jquery.com/jquery-1.10.1.min.js"></script> </head> <body> <p align="center"><input type="button" id="share_screen" value="Share screen"/></p> <p align="center"><video id="video"/></p> </body> </html>
Now we can add handler for click on share button, that will request screen media stream (using the code above) and play it in our video element:
<script language="javascript”> navigator.getUserMedia = navigator.webkitGetUserMedia || navigator.getUserMedia; $('#share_screen').click(function() { navigator.getUserMedia({ audio: false, video: { mandatory: { chromeMediaSource: 'screen', maxWidth: 1280, maxHeight: 720 }, optional: [] } }, function(stream) { document.getElementById(‘video’).src = window.URL.createObjectURL(stream); $('#share_screen').hide(); }, function() { alert('Error, my friend. Screen stream is not available. Try in latest Chrome with Screen sharing enabled in about:flags.'); } ) }) </script>
So instead of adding a stream to peer connection, we’re setting it as video surce.
And…That’s it! Here’s a page that we’ve made:
<html> <head> <title>Screen sharing example</title> <script src="https://code.jquery.com/jquery-1.10.1.min.js "></script> </head> <body> <p align="center"><input type="button" id="share_screen" value="Share screen"/></p> <p align="center"><video id="video" autoplay/></p> <script language="javascript"> navigator.getUserMedia = navigator.webkitGetUserMedia || navigator.getUserMedia; $('#share_screen').click(function() { navigator.getUserMedia({ audio: false, video: { mandatory: { chromeMediaSource: 'screen', maxWidth: 1280, maxHeight: 720 }, optional: [] } }, function(stream) { document.getElementById('video').src = window.URL.createObjectURL(stream);; $('#share_screen').hide(); }, function() { alert('Error, my friend. Screen stream is not available. Try in latest Chrome with Screen sharing enabled in about:flags.'); } ) }) </script> </body> </html>
When the user clicks on the “Share screen” button, click handler will request media stream and display it in video player if the permission is granted.
Good post Tsahi – way to keep to WebRTC at the forefront of the industry.
Thanks @kkooler – whew, that’s heavy stuff for a UCC Sales Exec like me. BUT just right for the developer folks. A very cool recipe for them.
I have enable the ‘screen capture support..’ flag and run the code in chrome but getting an error that’screen stream is not available’…..how to handle this?
waiting for your reply
You must control that you have a https connections…. It is mandatory!
Using WebRTC we had done upto video conferencing and now we are trying to work on video sharing to add to our present tool. we need your help. soon and we are expecting some video demonstration link for clear understanding…I am a beginner and student …
Naveen,
Your best bet would be to checkout stackoverflow and https://webrtcHacks.com for such information and assistance.
SOrry sir, i am not able open that link of screen sharing example. will you please activate it send me another link of it. And futher, sir i want to know how broadcast cam over web using wertc. if you help me in this it will me very helpful to me. thanks.
The example code link is a 404: https://dl.dropboxusercontent.com/u/178301/screen_sharing_example.html
Thanks for the comment.
It is an old post that probably requires an update. For now, I’ll indicate at the top of it that this has issues.
It seens code is not available It shows 404. Can you please give me updated link
nice post
As I stated, the piece of code is no longer relevant, so better look at other resources available online. I might revisit this one and rewrite it at some point.
Any idea how to allow screen sharing with voice recording at the same time? What I have done is I was able to do screen sharing but no audio.
Jonathan, getDisplayMedia (which replaces getUserMedia for screen sharing) also supports audio:true in its constraints.
Is it possible to develop screen capture using Jquery
Not sure I follow the question…
hi can we hide screen sharing prompt and capture entire screen automatically. Thanks
Not really. This is considered a privacy/security measure so not something you can skip.
You can remove it in an Electron app by modifying the source code and you can also automatically approve and remove the prompt if you are running Chrome from CLI and can add whatever you want to the parameters you start it with (this one is used for test automation purposes).
Hello,
I am planing to implement screen sharing functionality and it is working fine in desktop browser but it is not working on android browser as "getDisplayMedia" does not support mobile browser (https://developer.mozilla.org/en-US/docs/Web/API/MediaDevices/getDisplayMedia). Can some one guide me what is alternate way to achieve that
discuss-webrtc and stackoverflow might be better suited for these troubleshooting working/not working questions than a comment on this blog.